Token Based Authentication
Token-based authentication is a popular method for securing APIs and applications. In this guide, we'll explore how it works and why it's a go-to solution for developers looking to enhance security without sacrificing user experience.
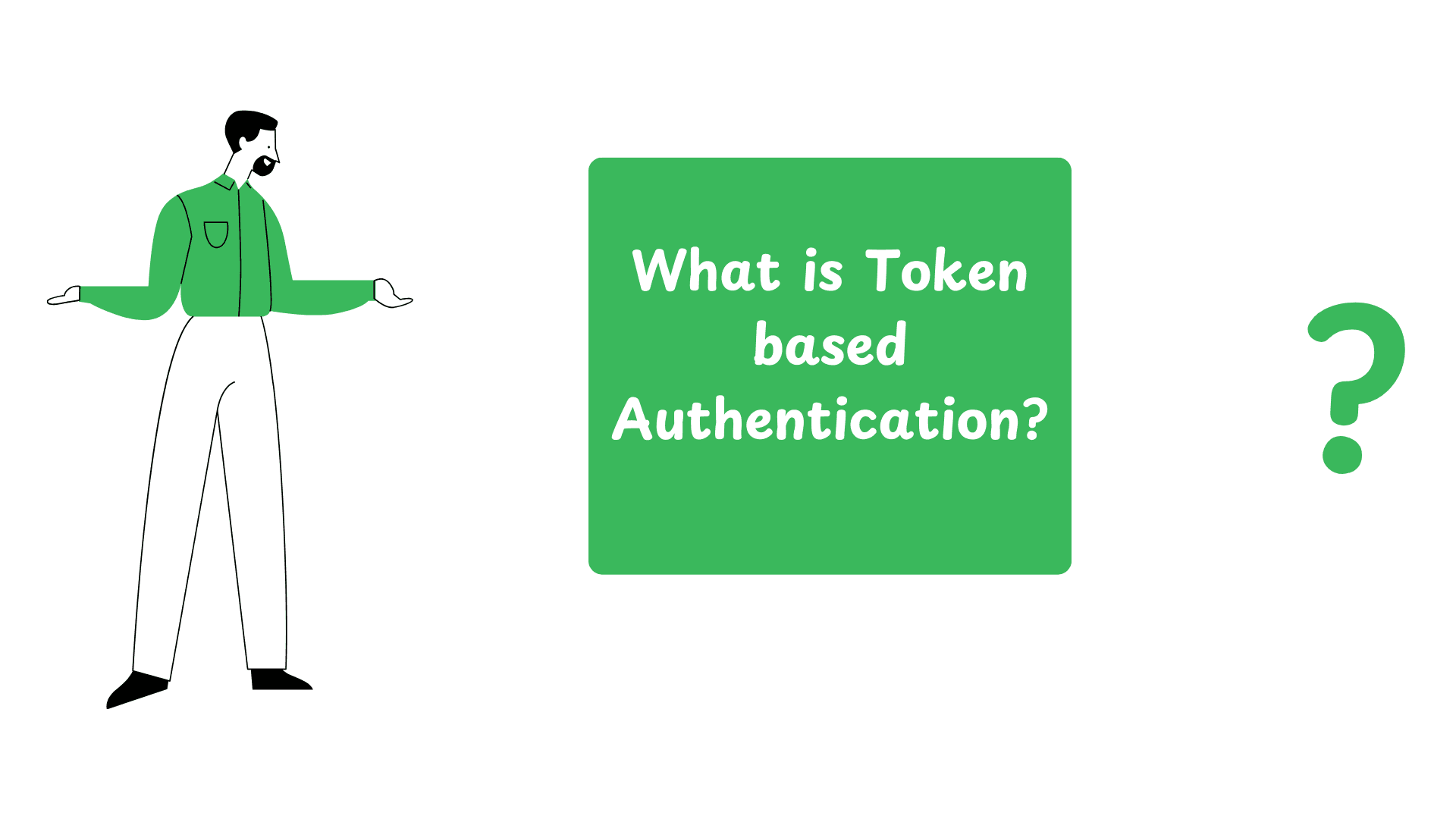
Token Based Authentication
Token-based authentication is a widely adopted method for secure access in API-driven architectures, providing robust solutions for web and mobile security.
This authentication protocol allows users to verify their identity using authentication tokens, which grant access to specific resources without constantly resending sensitive information like passwords.
As a key element in modern authentication protocols, token-based systems enhance security by reducing vulnerabilities in both web and mobile environments. This method is particularly well-suited for API communication, offering a scalable and efficient way to secure data exchanges.
In this article, we will explore all aspects of token-based authentication, including how token based authentication works, the types of tokens used in authentication, and the key benefits it offers for both developers and users. By the end, you’ll understand why token-based authentication is crucial, and how it contributes to stronger web security and efficient authentication protocols.
How Does Token-Based Authentication Work?
Token-based authentication is a process where a user verifies their identity through tokens, which are passed with each request to access secure resources. This method is widely used in modern web and mobile applications due to its scalability, security, and ease of use. Here’s a step-by-step explanation of how token-based authentication functions:
User Submits Credentials
The process starts when the user inputs their login credentials, usually a username and password, through a client application (e.g., a web or mobile app). These credentials are sent securely to the Authorization Server via an HTTPS request. This step allows the user to prove their identity to the server, initiating the authentication process.
Authorization Server Validates Credentials
Upon receiving the credentials, the Authorization Server checks whether the provided information matches what is stored in its database. This step includes verifying the user’s identity and checking whether the account is valid (e.g., not locked or disabled). If the credentials are correct and the user is authenticated successfully, the server generates an Access Token and a Refresh Token.
These tokens are then sent back to the client to be used in future interactions. The Access Token is typically short-lived, while the Refresh Token has a longer lifespan, allowing the client to request new access tokens without needing to authenticate the user again.
Client Stores Tokens:
Once the Access Token and Refresh Token are received, the client securely stores them. For web applications, the Access Token is commonly stored in browser local storage or session storage, while in mobile applications, it is stored in secure storage like the Keychain (iOS) or Keystore (Android).
The Access Token will be used to authenticate future requests to protected resources, while the Refresh Token is reserved for obtaining a new access token when the current one expires. Secure storage of these tokens is crucial to prevent unauthorized access to protected resources.
Client Sends Token to Resource Server:
When the user needs to access a protected resource, such as user-specific data or a restricted API, the client app sends a request to the Resource Server. Along with the request, the client attaches the Access Token in the HTTP headers. This is usually done with the following format:
Authorization: Bearer Access_Token
The Access Token acts as proof that the user has been authenticated and is allowed to access the requested resource.
Resource Server Validates Token:
Upon receiving the request, the Resource Server extracts the Access Token from the request headers and validates it. The server checks the token’s integrity, expiration, and whether it was signed by the trusted Authorization Server. If the token is valid, the Resource Server proceeds to fulfill the request.
If the token is invalid, expired, or tampered with, the server rejects the request, typically returning an HTTP status code 401 (Unauthorized) or 403 (Forbidden), prompting the client to either re authenticate or refresh the token.
Accessing Resources
If the Access Token is valid, the Resource Server processes the request and provides access to the requested resource. This could involve retrieving user data, allowing actions like file uploads, or accessing sensitive information. The interaction between the client and the resource server is now secured by the token, without requiring the user to submit their credentials again, streamlining the experience.
Token Expiry and Refresh
Access tokens typically have a limited lifespan to minimize the risks associated with token theft. Once the Access Token expires, the client cannot access protected resources using the expired token. However, instead of asking the user to log in again, the client can use the Refresh Token to request a new Access Token from the Authorization Server.
The server validates the Refresh Token, and if it's valid, issues a new Access Token (and possibly a new Refresh Token). This process ensures a smooth user experience, allowing continuous access without repeated logins, while maintaining security by rotating tokens.
What are the Types of Tokens Used in Token Based Authentication?
Token-based authentication relies on different types of tokens to grant secure access to various resources. The most common token types are JSON Web Tokens (JWT), OAuth tokens, and SAML tokens. Each serves a unique role in authentication processes, and choosing the right one depends on the specific use case.
JSON Web Tokens (JWT)
JSON Web Tokens (JWT) are compact, URL-safe tokens widely used for API authentication. A JWT consists of three parts: a header, a payload, and a signature. The payload contains claims, which are statements about the user or other data, and the token is signed to ensure that it hasn't been tampered with.
JWTs are stateless, meaning the server does not need to store any session data, which makes them ideal for distributed systems and mobile apps. Their ease of use and ability to carry information securely between parties make them one of the most common token types in modern web applications.
OAuth Tokens
OAuth tokens are primarily used in API authentication when delegated access to resources is required. OAuth is an authorization framework that allows users to grant third-party services limited access to their resources without exposing their credentials. OAuth tokens come in two types: access tokens and refresh tokens.
Access tokens allow the client to access specific resources for a limited time, while refresh tokens can be used to obtain new access tokens without re-authentication. OAuth tokens are commonly used for social logins, third-party integrations, and situations where secure, temporary access to APIs is needed.
SAML Tokens
SAML (Security Assertion Markup Language) tokens are XML-based tokens used for Single Sign-On (SSO) in enterprise environments. SAML tokens allow users to authenticate once with an identity provider (IdP) and access multiple services without needing to re-enter credentials.
This is particularly useful for managing user access across a variety of applications in corporate settings. SAML tokens offer strong security features, making them a preferred choice for large organizations looking to streamline authentication across several internal and external services.
Differences Between Token Types and Best Use Cases
Like we said earlier, each token type—JWT, OAuth tokens, and SAML tokens—has unique strengths and is best suited for specific authentication scenarios. JSON Web Tokens (JWTs), OAuth tokens, and Security Assertion Markup Language (SAML) tokens all provide secure access, but their use cases vary depending on factors like statelessness, delegated access, and Single Sign-On (SSO) requirements.
JWTs are ideal for stateless applications, where the server does not need to store user sessions. They are widely used in mobile and API-driven applications due to their compact size and ability to be transmitted easily between the client and server. JWTs store user information directly in the token itself, which allows the system to verify user identity without querying a database for session data.
This leads to faster authentication processes and makes JWTs especially beneficial for distributed systems and microservices architectures, where scalability and performance are top priorities.
OAuth tokens, on the other hand, excel in scenarios where third-party services need delegated, temporary access to user resources. OAuth is handy for social media logins, where an application requests limited user data access without exposing sensitive credentials. OAuth generates access tokens and refresh tokens, which allow third-party applications to access specific resources on behalf of the user.
These tokens are essential in scenarios where secure, scoped access is necessary, making OAuth a popular choice for API integrations and services like Google, Facebook, or Twitter logins.
SAML tokens are best suited for enterprise environments where Single Sign-On (SSO) is required. SAML allows users to authenticate once and access multiple applications without having to log in separately to each one. This is particularly useful for organizations with numerous internal or third-party services that employees need to access.
SAML’s XML-based protocol securely transfers authentication data between an identity provider (IdP) and service providers (SPs), ensuring seamless access across platforms. Its robust security features and SSO capabilities make SAML a popular choice for large organizations and enterprise-grade applications that need to manage user access efficiently.
What Are the Differences Between Token Based Authentication and Session Based Authentication?
Token-based and session-based authentication are two distinct approaches to handling user sessions, and understanding their differences is essential for choosing the right one based on your application’s needs. Below, we break down the key differences between these two methods.
- Storage Location
Session-based authentication stores session information on the server side, typically in memory or a database. Each user is assigned a unique session ID, which is then used to retrieve the relevant session data.
Token-based authentication, on the other hand, stores the authentication information on the client side. The server generates a token (often a JSON Web Token or JWT) containing user information and signs it. This token is then stored by the client, usually in local storage or a cookie.
- Stateful vs Stateless
Session-based authentication is stateful. The server needs to keep track of active sessions, which can be challenging in distributed systems or when scaling horizontally.
Token-based authentication is stateless. Each request from the client to the server is accompanied by the token, which contains all the necessary information. This makes it easier to scale and allows for more flexibility in distributed systems.
- Scalability and Performance
Due to its stateless nature, token-based authentication generally offers better scalability and performance, especially in distributed systems. Servers don't need to query a centralized session store for every request, reducing latency and database load.
Session-based authentication can face challenges when scaling, as maintaining session consistency across multiple servers can be complex. It may require additional infrastructure like shared caching systems to manage sessions effectively.
Real-World Examples Where Token-Based Authentication Outperforms Session-Based Authentication
While much ink has been spilled over which authentication method is better, no one can deny that there are specific cases where token-based authentication outshines session-based authentication.
For instance, token-based authentication is the preferred choice in modern applications that adopt a microservices architecture due to its stateless nature. Each service often operates independently in a microservices environment and can scale separately, and since token-based systems do not require server-side session storage, they simplify the authentication process across multiple microservices.
For example, an e-commerce platform using various services for payments, inventory, and user profiles can rely on token-based authentication to securely verify users without replicating session data across each service. This reduces overhead and increases scalability, making token-based systems more efficient in distributed architectures.
Token-based authentication also outperforms session-based authentication in mobile applications and SPAs, where persistent client-server communication is crucial. These applications often rely on REST APIs for backend communication, and stateless tokens (like JWTs) ensure that the client can maintain a session without needing server-side state.
In mobile banking apps or fitness tracking apps, users can stay authenticated as long as the token remains valid, without requiring the server to store or track session IDs. This leads to a more seamless user experience and allows mobile apps to operate more smoothly, especially when the client needs to access different services.
Finally, token-based authentication is highly advantageous in serverless architectures and cloud platforms like AWS Lambda or Azure Functions. These environments do not maintain state between requests, making it difficult to use session-based authentication, which requires tracking user sessions on the server. Token-based authentication, being stateless, fits perfectly into this model by allowing each function or service to validate tokens without storing session data.
For example, token-based authentication enables secure, scalable interactions across multiple services without managing session replication or storage in serverless applications that provide on-demand services like file storage or video streaming.
What Are the Security Best Practices for Token Based Authentication?
Token-based authentication is highly effective, but it comes with security challenges that require careful handling. These challenges may seem emense, but they can be overcome by implementing the following best practices to ensure that token-based authentication systems remain secure.
- Token Encryption and Signing
Encrypting and signing tokens are critical to ensuring the security and integrity of the data they contain. Signing tokens with a secret key or public/private key pair allows the server to verify that the token hasn’t been tampered with during transmission.
Encryption ensures that sensitive information within the token, such as user data, is protected from attackers. It’s essential to use strong cryptographic algorithms such as HMAC SHA256 for signing and secure encryption methods like RSA or AES to prevent token forgery and data leaks.
- Short Token Expiration Times
Using short token expiration times is one of the most effective ways to minimize security risks. By limiting how long a token remains valid, you reduce the window of opportunity for attackers to exploit a stolen or compromised token.
Tokens should ideally expire within minutes or hours, depending on the application’s security needs. For prolonged sessions, refresh tokens can be used to obtain new access tokens without requiring the user to reauthenticate constantly.
- Secure Implementation of Refresh Tokens
Refresh tokens provide a way to maintain user sessions without requiring frequent logins, but they must be implemented securely. Since they have longer lifetimes than access tokens, they should be stored in a highly secure manner, such as using HttpOnly and Secure cookies.
Additionally, security tokens should be rotated with each request to minimize their reuse and limit the risk of token theft. Properly managing the issuance and expiration of these tokens is key to preventing unauthorized access and further securing a user's identity.
- Token Revocation and Invalidation
It is important to have a system in place for revoking and invalidating tokens, especially in cases of security breaches or user logouts. Token revocation mechanisms allow you to invalidate a token before its natural expiration time. This can be particularly useful if a token is compromised, giving administrators the ability to revoke the token’s access rights immediately.
Maintaining a token blacklist and checking against it for each request helps ensure that revoked tokens cannot be reused.
- Secure Token Storage
Avoid storing tokens in insecure locations, such as local storage, as these are vulnerable to cross-site scripting (XSS) attacks. Instead, use HttpOnly and Secure cookies to store tokens, as these attributes prevent client-side JavaScript from accessing the cookies and ensure the token is transmitted only over HTTPS.
Another option is to use session storage, which is generally safer than local storage, as the token is automatically deleted when the session ends, reducing the risk of token exposure.
Pro tip: You should also run periodic token tests to verify that your tokens are working correctly By following these best practices, you can significantly enhance the security of your token-based authentication system, protecting user's identity against common vulnerabilities and ensuring secure, reliable user authentication.
Conclusion
Token authentication offers a robust, scalable, and efficient method for securing access to resources in modern API-driven architectures. Whether using JSON Web Tokens (JWT), OAuth tokens, or SAML tokens, the flexibility and stateless nature of tokens allow applications to handle user sessions more efficiently, especially in distributed systems, mobile apps, and cloud environments.
Token authentication simplifies user verification while enhancing performance and scalability by eliminating the need for server-side session management.
However, it is crucial to implement security best practices to ensure the integrity of token authentication systems. Encryption and signing, short token expiration times, token revocation, and proper token storage are all essential steps in mitigating potential risks.