Open-source: Implement Role Based Access Management with Permify React Role
In this tutorial we will share an effective approach to implementing RBAC in React applications with React Role, which is an open-source RBAC solution from Permify.
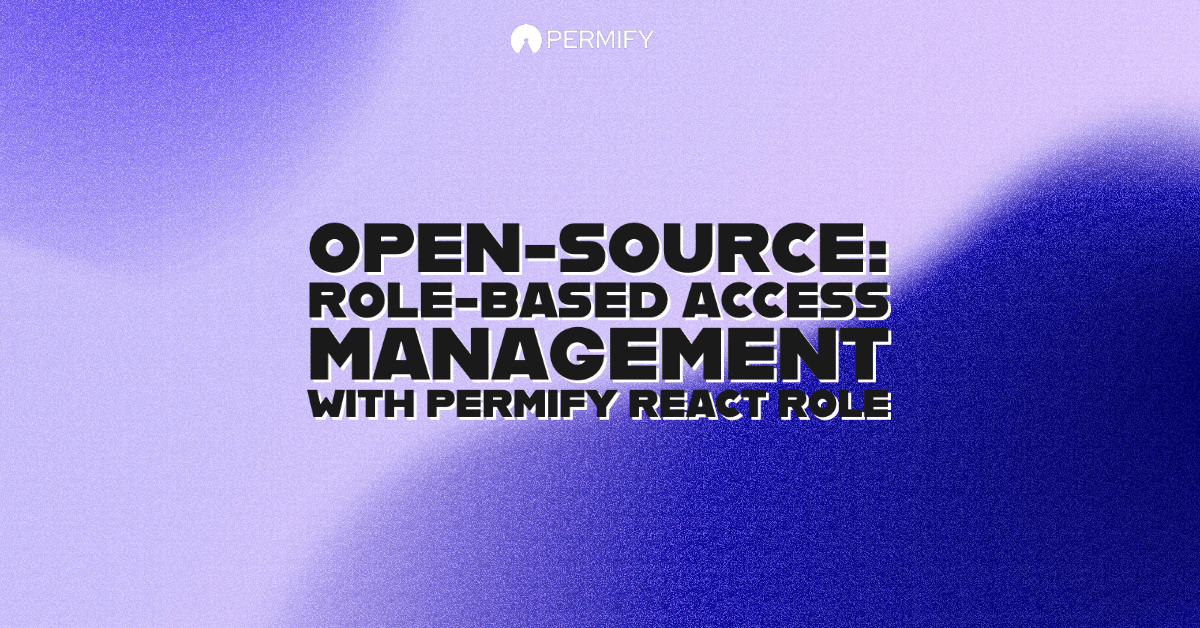
summary: 'In this tutorial we will share an effective approach to implementing RBAC in React applications with React Role, which is an open-source RBAC solution from Permify.'
Introduction
Authorizations have always been an issue in many developers’ minds. Some build simple databases that route a couple of roles, and others configure external services.
But there is a well-established best practice for authorizations today. During the development, it should be well-structured and well-designed.
Otherwise, you’re doomed to technical debt.
For instance; let’s take RBAC into account. RBAC means Role-based Access Control where you basically check access requests based on roles and permissions.
Although RBAC does provide advantages to the developers at many points, it can also create difficulties for the developers very early on
On an RBAC system, depending on the roles and permissions, we decide which parts of the application will be accessible or restricted for the user.
Usually, this process results in many nested if and else statements,
When you consider a small-scale non-complex application, this if and else statements works just fine. But as things grow, and you acquire a wider audience, you encounter problems with your decision model and performance.
At this point, adding new if and else statements will be added on top of the already developed structure, which will make it harder to ship new features, debug and refactor the code which leads to inevitable technical debt.
In this tutorial, I will share an effective approach to implement RBAC in React applications with React Role which is an open source RBAC solution from Permify.
React Role contains components, hooks and helper methods that provides many advantages in terms of access management by controlling the role and permissions of the user without any backend connection.
I’ll develop a CRM-kinda application, to introduce the basis of React Role. I’ll define and set mock that for users, users’ roles and users’ permissions in order to roll access checks.
Let’s start!
Step 1: Setup the React project
From your Terminal or Command Prompt, execute the following command to create a new React project:
npx create-react-app react-role-demo
You can get more information about creating a new React application by checking the React documentation.
After creating the applicaiton, clear out in order to start fresh. When you done, your folder structure, App.js and index.js files should look like this;
App JS
function App() {
return <div>React Role Demo</div>;
}
export default App;
Index JS
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(<App />);
Step 2: Build UI
I used the Ant Design library for the interface. For the ones who are new to React, it is possible to use many pre-build components with Ant Design. If you wish, you can use a different UI library in this step.
But the following steps will be based on Ant Design.
To use Ant Design in your project, firstly execute the following command in your project folder to install Ant Design. You can get more information about Ant Design by checking its documentation and components.
yarn add antd
Our application will have two different pages. One of them is the login page and the other one is the home page.
To briefly explain, users will be able to login to our application by entering their email on the login page. Thus, we we will obtain what roles and permissions the user has with the email information that was given us.
In our home page, we’ll have a table with the contact information. And 2 consecutive buttons as seen in following image. The red one is for log out, and the purple one is for creating a contact.
But we’ll restrict this contact create button only for the certain peoples' use.
End of the tutorial, I will share the GitHub repository link of the application. So if you want to take a look at the source code, you can visit the given link.
Step 3: Create Sample Users
Within the application, there will be 3 different user roles such as admin, editor and agent. As I mentioned, we want to restrict access of Create Contact button depending on these rules.
We’ll make Create Contact button only visible and available for the users who have the admin or editor role.
And thise button will be restirected for agent roles.
But besides roles, we want to check access for these button via the users’ permissions. If the user has “contact-create” permissions, we’ll allow them to access this button regardless their roles.
In line with this plan, let’s create sample users as follows:
[
{
"id": "1fbb807d972847cbb65096a22403bd2f",
"email": "louise@permify.co",
"name": "Louise Walker",
"role": "admin",
"permission": ["project-index", "project-create", "project-delete"]
},
{
"id": "b108ceae1cfb46dfb34e3aa7c9f28606",
"email": "miguel@permify.co",
"name": "Miguel Jensen",
"role": "editor",
"permission": ["content-index", "content-create", "content-delete"]
},
{
"id": "c2349776bd364fac9debf397e3f4538a",
"email": "greg@permify.co",
"name": "Greg Howell",
"role": "agent",
"permission": ["contact-index"]
},
{
"id": "73b612c40a2044718123d476e45a2245",
"email": "leonard@permify.co",
"name": "Leonard Wade",
"role": "agent",
"permission": ["contact-index","contact-create", "contact-delete"]
},
{
"id": "9455a30af8e141ba905a27e7855babde",
"email": "alex@permify.co",
"name": "Alex Collins",
"role": "agent",
"permission": ["contact-index"]
}
]
Step 4: Add React Role
After creating sample users, we need to install the React Role library to set up access management depending on the user's role and permissions. To install React Role execute the following command in your project folder.
yarn add @permify/react-role
After installing React Role, we need to wrap all the structures in the App.js file that we want to control access check with the PermifyProvider component.
At the same time, in this App.js file, we will check the user information that we have saved to localStorage on the Login page in order to see which user is logged into the system and send it to the Home page as props.
...
import { PermifyProvider } from "@permify/react-role";
function App() {
const [user, setUser] = useState(null);
useEffect(() => {
setUser(JSON.parse(localStorage.getItem("user")));
}, []);
return (
<PermifyProvider>
{user === null ? <Login /> : <Home user={user} />}
</PermifyProvider>
);
}
export default App;
For the Home page, as I said before, the Create Contact button must be visible if the user has admin role, editor role, or has contact-create permission.
To control the user’s role and permission, we need to use the HasAccess component in the React Role library.
HasAccess component takes 3 different props to check whether a user has access to an element or not.
- Roles: Set the availability of a component by Roles.
- Permissions: Set the availability of a component by Permissions.
- renderAuthFailed: Set what should be rendered instead of this button if it is not accessible.
...
import { HasAccess } from "@permify/react-role";
...
function Home(props) {
...
return (
...
<HasAccess
roles={["admin", "editor"]}
permissions="contact-create"
renderAuthFailed={null}
>
<div className="button create-btn">Create Contact</div>
</HasAccess>
...
);
}
export default Home;
In order to make the source code more readable and easy to understand, some parts of the code are not included. In which there were fake data requests that are used for the showing in the table and table’s own setup. If you want to check whole source code, you can visit the GitHub repository.
Step 5: Test It Out
It is time to test our application and take a closer look at the power of the HasAccess component.
First let’s sign in as Louise Walker with louise@permify.co email address who has admin role. Hence Louise will be able to see the Create Contact button.
This will be the same as for the editor role because we send roles props as [“admin”, “editor”] to the HasAccess component.
Now, let’s sign in as Greg Howell who has an agent role. Due to his role, Create Contact button will invisible, because renderAuthFilled props is setted as {null}.
At last, let’s sign in as Leonard Wade with role of agent. But, Leonard also has contact-create permission.
Normally, Leonard should not see the Create Contact button because of the agent role. But thanks to the contact-create permission, he will be able to access the Contact Create button because the permission props is set as “contact-create”.
Conclusion
For this tutorial, I tried to keep everything simple. And stay in the epicentral points of the React Role library.
If you have any questions or doubts, please do not hesitate to ask at ege@permify.co . Also, here's the source code, and the open-source library;
Source Code: https://github.com/erenolgun/react-role-demo Open-source Library: https://github.com/Permify/react-role