How to Implement RBAC in Next.js in Mere Minutes with Permify
This guide will walk you through the process of setting up role based authorization in your Next.js application using Permify, taking you from zero to a basic, functional implementation in mere minutes.
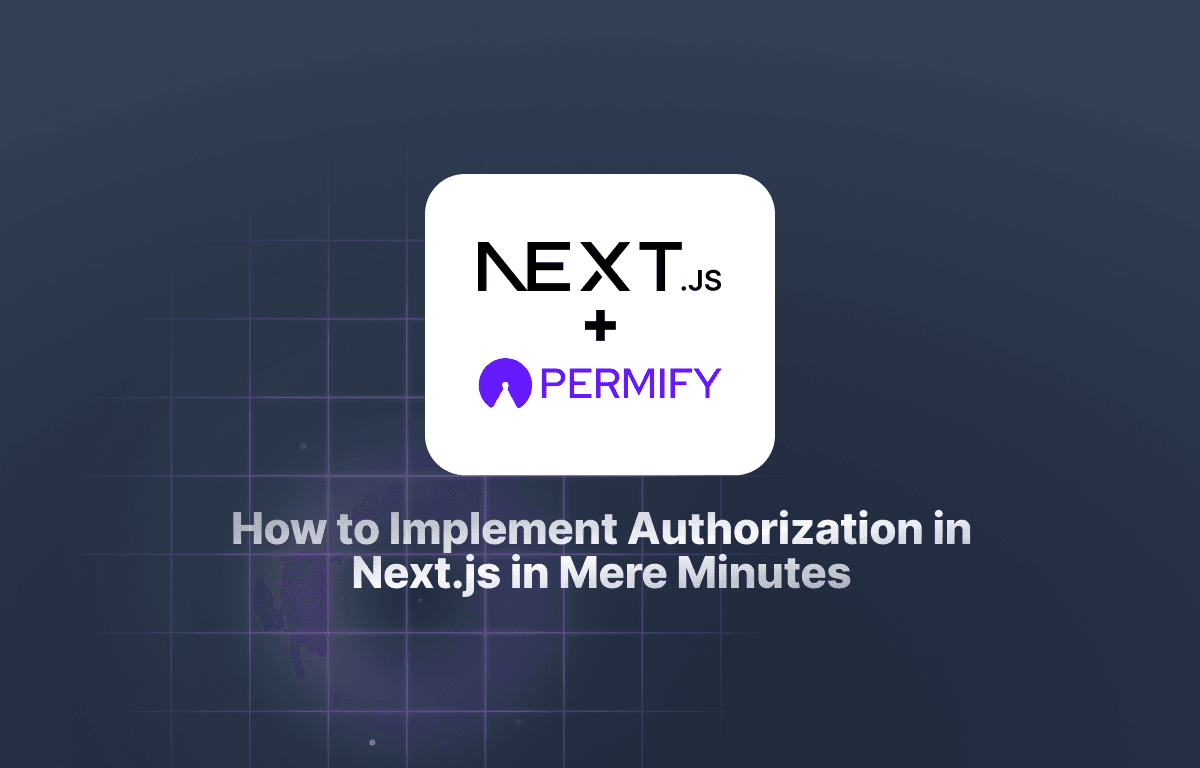
Prerequisites
- Basic Knowledge of React, and Next.js
- Basic Understanding of REST APIs
- A code editor (Preferably Vscode)
- Node.js Installed
- Cors Unblock - A chrome extension that allows cors in the browser.
Step 1: Setting Up Next.js Application
We will create a simple Next.js fullstack app that allows different users to login account based to their roles and permission using Permify to implement access control.
Project structure
my-permify-nextjs-app/
├── public/
| ├── next.svg
│ └── vercel.svg
├── app/
│ ├── api
│ │ └── permission.js
| ├── page.js
│ ├── admin.js
│ ├── error.js
│ ├── index.js
| ├── member.js
│ ├── manager.js
| ├── layout.js
| ├── favicon.ico
│ └── global.css
├── app.js
├── app-relationship.js
├── app-tenant.js
├── app-schema.js
├── package.json
├── tailwind.config.js
├── jsconfig.json
├── package-lock.json
├── postcss.config.mjs
├── .gitignore
└── next.config.mjs
Building the Frontend
1. Create a new Next.js project:
npx create-next-app@latest my-permify-nextjs-app
Then
cd my-permify-nextjs-app
2. Install dependencies:
npm install @permify/permify-node express react-chartjs-2 chart.js cors body-parser
3. Replace your current page.js
code with the code below.
"use client" // To specify client side component
import React, { useState } from 'react';
import { useRouter } from 'next/router';
export default function IndexPage() {
const router = useRouter();
const [userId, setUserId] = useState('');
// Manual configuration for the API port
const apiPort = 3000;
const handleLogin = async (role) => {
try {
if (!userId) {
alert('User ID is missing. Please enter your User ID.');
return;
}
// send the role and userId to the backend for permission check
const response = await fetch(`http://localhost:${apiPort}/${role}/${userId}`);
const data = await response.json();
if (data.message === 'You are authorized!') {
router.push(`/${role}`); // sends the authorized user to the authorized role page
} else {
router.push('/error'); // sends unauthorized user to an error page.
}
} catch (error) {
console.error('Error during login:', error);
router.push('/error');
}
};
const handleUserIdChange = (e) => {
setUserId(e.target.value);
};
return (
<div className="flex min-h-screen items-center justify-center bg-gray-100">
<div className="w-full max-w-md p-6 bg-white rounded-md shadow-lg shadow-blue-500/50">
<div className="text-center mb-6"> {/* Add text-center for center alignment and mb-6 for bottom margin */}
<h2 className="text-2xl font-semibold text-gray-900">Welcome User! Please Login</h2> {/* Add heading styles */}
</div>
<div className="mb-4">
<input
type="text"
placeholder="Enter Your User ID"
value={userId}
onChange={handleUserIdChange}
className="w-full px-4 py-2 border rounded-md focus:outline-none focus:ring focus:ring-blue-500 text-gray-900" // Add text-gray-900 for black text
/>
</div>
<div className="flex justify-between space-x-2"> {/* Add space-x-2 for spacing between buttons */}
<button
onClick={() => handleLogin('admin')}
className="px-4 py-2 bg-blue-500 text-white rounded-md hover:bg-blue-600 focus:outline-none focus:ring focus:ring-blue-500 text-sm" // Reduce text size
>
Admin Login
</button>
<button
onClick={() => handleLogin('manager')}
className="px-4 py-2 bg-blue-500 text-white rounded-md hover:bg-blue-600 focus:outline-none focus:ring focus:ring-blue-500 text-sm" // Reduce text size
>
Manager Login
</button>
<button
onClick={() => handleLogin('member')}
className="px-4 py-2 bg-blue-500 text-white rounded-md hover:bg-blue-600 focus:outline-none focus:ring focus:ring-blue-500 text-sm" // Reduce text size
>
Member Login
</button>
</div>
</div>
</div>
);
}
This is the landing page for the app with a simple login built using Next.js and Tailwind CSS. It has a welcome message and an input field where users can enter their user Id
.
The page has three buttons for "Admin Login,
" "Manager Login,
" and "Member Login.
" When you click on any of these buttons, the code sends a GET request
to the backend API with the entered User ID, and the selected role (admin, member, or manager).
If the backend API confirms the user is authorized, it redirects the user to the corresponding page (dashboard, member, or manager). If the user is unauthorized, the user is redirected to an error page.
To see the login page, run this command;
npm run dev
4. Create these pages in app
directory:
admin.js:
This is the component for the admin dashboard page, accessible only to admins. The code below is a demo admin page, you can add it or use your own code.
import React from 'react';
import { useState, useEffect } from 'react';
import { Chart as ChartJS, CategoryScale, LinearScale, PointElement, LineElement, Title, Tooltip, Legend } from 'chart.js';
import { Line } from 'react-chartjs-2';
ChartJS.register(
CategoryScale,
LinearScale,
PointElement,
LineElement,
Title,
Tooltip,
Legend
);
function AdminContent() {
const [managers, setManagers] = useState([]);
const [members, setMembers] = useState([]);
const [chartData, setChartData] = useState(null);
useEffect(() => {
// Fetch or simulate data for managers and members
const fetchDemoData = async () => {
// Replace this with your actual data fetching logic
const demoManagers = [
{
name: 'Alice Johnson',
image: 'https://picsum.photos/200/300', // Placeholder image
email: 'alice.johnson@example.com',
joinedDate: '2023-08-15'
},
{
name: 'Bob Williams',
image: 'https://picsum.photos/200/300', // Placeholder image
email: 'bob.williams@example.com',
joinedDate: '2023-09-20'
},
// ... add more managers
];
const demoMembers = [
{
name: 'Carol Brown',
image: 'https://picsum.photos/200/300', // Placeholder image
email: 'carol.brown@example.com',
joinedDate: '2023-10-05'
},
{
name: 'David Garcia',
image: 'https://picsum.photos/200/300', // Placeholder image
email: 'david.garcia@example.com',
joinedDate: '2023-11-10'
},
// ... add more members
];
// Chart data (replace with your actual data)
const chartData = {
labels: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun'],
datasets: [
{
label: 'Sales',
data: [30, 50, 60, 40, 70, 90],
borderColor: 'rgb(53, 162, 235)',
tension: 0.4,
},
],
};
setManagers(demoManagers);
setMembers(demoMembers);
setChartData(chartData);
};
fetchDemoData();
}, []);
return (
<div className="bg-gray-100 min-h-screen">
<div className="container mx-auto p-4">
<h1 className="text-3xl font-bold text-gray-800 mb-4">Admin Dashboard</h1>
<div className="grid grid-cols-1 md:grid-cols-2 lg:grid-cols-3 gap-4">
{/* Manager List */}
<div className="bg-white rounded-md shadow-md p-4">
<h2 className="text-xl font-semibold text-gray-800 mb-2">Managers</h2>
<ul className="list-none">
{managers.map((manager) => (
<li key={manager.email} className="flex items-center mb-2">
<img
src={manager.image}
alt={manager.name}
className="w-10 h-10 rounded-full mr-2"
/>
<div>
<span className="font-medium">{manager.name}</span>
<br />
<span className="text-gray-600">{manager.email}</span>
<br />
<span className="text-gray-600">Joined: {manager.joinedDate}</span>
</div>
</li>
))}
</ul>
</div>
{/* Member List */}
<div className="bg-white rounded-md shadow-md p-4">
<h2 className="text-xl font-semibold text-gray-800 mb-2">Members</h2>
<ul className="list-none">
{members.map((member) => (
<li key={member.email} className="flex items-center mb-2">
<img
src={member.image}
alt={member.name}
className="w-10 h-10 rounded-full mr-2"
/>
<div>
<span className="font-medium">{member.name}</span>
<br />
<span className="text-gray-600">{member.email}</span>
<br />
<span className="text-gray-600">Joined: {member.joinedDate}</span>
</div>
</li>
))}
</ul>
</div>
{/* Demo Chart */}
{chartData && (
<div className="bg-white rounded-md shadow-md p-4">
<h2 className="text-xl font-semibold text-gray-800 mb-2">Sales Trend</h2>
<Line data={chartData} />
</div>
)}
</div>
{/* Additional content area (e.g., recent activity, reports, etc.) */}
{/* ... */}
</div>
</div>
);
}
export default AdminContent;
manager.js:
This is the component for the manager page, accessible to managers and admins. The code below is a demo manager page, you can add it or use your own code.
import React from 'react';
import { useState, useEffect } from 'react';
import { Chart as ChartJS, CategoryScale, LinearScale, PointElement, LineElement, Title, Tooltip, Legend } from 'chart.js';
import { Line } from 'react-chartjs-2';
ChartJS.register(
CategoryScale,
LinearScale,
PointElement,
LineElement,
Title,
Tooltip,
Legend
);
function ManagerContent() {
const [members, setMembers] = useState([]);
const [chartData, setChartData] = useState(null);
useEffect(() => {
// Fetch or simulate data for members
const fetchDemoData = async () => {
// Replace this with your actual data fetching logic
const demoMembers = [
{
name: 'Carol Brown',
image: 'https://picsum.photos/200/300', // Placeholder image
email: 'carol.brown@example.com',
joinedDate: '2023-10-05'
},
{
name: 'David Garcia',
image: 'https://picsum.photos/200/300', // Placeholder image
email: 'david.garcia@example.com',
joinedDate: '2023-11-10'
},
// ... add more members
];
// Chart data (replace with your actual data)
const chartData = {
labels: ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun'],
datasets: [
{
label: 'Tasks Completed',
data: [25, 35, 45, 30, 50, 60],
borderColor: 'rgb(53, 162, 235)',
tension: 0.4,
},
],
};
setMembers(demoMembers);
setChartData(chartData);
};
fetchDemoData();
}, []);
return (
<div className="bg-gray-100 min-h-screen">
<div className="container mx-auto p-4">
<h1 className="text-3xl font-bold text-gray-800 mb-4">Manager Dashboard</h1>
<div className="grid grid-cols-1 md:grid-cols-2 lg:grid-cols-3 gap-4">
{/* Member List */}
<div className="bg-white rounded-md shadow-md p-4">
<h2 className="text-xl font-semibold text-gray-800 mb-2">Members</h2>
<ul className="list-none">
{members.map((member) => (
<li key={member.email} className="flex items-center mb-2">
<img
src={member.image}
alt={member.name}
className="w-10 h-10 rounded-full mr-2"
/>
<div>
<span className="font-medium">{member.name}</span>
<br />
<span className="text-gray-600">{member.email}</span>
<br />
<span className="text-gray-600">Joined: {member.joinedDate}</span>
</div>
</li>
))}
</ul>
</div>
{/* Demo Chart */}
{chartData && (
<div className="bg-white rounded-md shadow-md p-4">
<h2 className="text-xl font-semibold text-gray-800 mb-2">Task Completion</h2>
<Line data={chartData} />
</div>
)}
{/* Demo Card */}
<div className="bg-white rounded-md shadow-md p-4">
<h2 className="text-xl font-semibold text-gray-800 mb-2">Recent Activity</h2>
<div className="flex flex-col items-center">
<img
src="https://picsum.photos/200/200"
alt="Activity Image"
className="rounded-md w-48 h-48 mb-2"
/>
<p className="text-gray-600 text-center">
Lorem ipsum dolor sit amet, consectetur adipiscing elit.
Nulla facilisi. Donec pulvinar, justo sit amet scelerisque
bibendum, quam libero condimentum elit, id rhoncus lectus
purus ac neque.
</p>
</div>
</div>
</div>
{/* Additional content area (e.g., reports, tasks, etc.) */}
{/* ... */}
</div>
</div>
);
}
export default ManagerContent;
member.js:
This is the component for the member page, accessible to members, and managers. The code below is a demo member page, you can add it or use your own code.
import React from 'react';
import { useState, useEffect } from 'react';
function MemberPage() {
const [posts, setPosts] = useState([]);
const [profile, setProfile] = useState(null);
useEffect(() => {
// Fetch or simulate data for posts and profile
const fetchDemoData = async () => {
// Replace this with your actual data fetching logic
const demoPosts = [
{
image: 'https://picsum.photos/600/400',
caption: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.'
},
{
image: 'https://picsum.photos/600/400',
caption: 'Nulla facilisi. Donec pulvinar, justo sit amet scelerisque bibendum, quam libero condimentum elit.'
},
{
image: 'https://picsum.photos/600/400',
caption: 'Nulla facilisi. Donec pulvinar, justo sit amet scelerisque bibendum, quam libero condimentum elit.'
},
{
image: 'https://picsum.photos/600/400',
caption: 'Nulla facilisi. Donec pulvinar, justo sit amet scelerisque bibendum, quam libero condimentum elit.'
}
// ... add more posts
];
const demoProfile = {
name: 'John Doe',
username: '@johndoe',
profileImage: 'https://picsum.photos/200/200',
bio: 'Hello! Welcome to the member page.',
bannerImage: 'https://picsum.photos/1200/400'
};
setPosts(demoPosts);
setProfile(demoProfile);
};
fetchDemoData();
}, []);
return (
<div className="bg-gray-100 min-h-screen">
{profile && (
<div className="container mx-auto">
{/* Banner Image */}
<div className="relative">
<img
src={profile.bannerImage}
alt="Banner"
className="w-full h-48 object-cover"
/>
<div className="absolute bottom-4 left-4 flex items-center">
<img
src={profile.profileImage}
alt={profile.name}
className="w-16 h-16 rounded-full"
/>
<div className="ml-4">
<h2 className="text-xl font-bold text-black">{profile.name}</h2>
<p className="text-black">{profile.username}</p>
</div>
</div>
</div>
{/* Profile Details */}
<div className="bg-white rounded-md shadow-md p-4 mt-4">
<p className="text-gray-900 text-center mb-2">{profile.bio}</p>
</div>
{/* Posts Grid */}
<div className="grid grid-cols-1 sm:grid-cols-2 md:grid-cols-3 lg:grid-cols-4 gap-4 mt-4">
{posts.map((post) => (
<div key={post.image} className="bg-white rounded-md shadow-md p-2">
<img
src={post.image}
alt="Post"
className="w-full h-48 object-cover rounded-md"
/>
<p className="text-gray-600 mt-2">{post.caption}</p>
</div>
))}
</div>
</div>
)}
</div>
);
}
export default MemberPage;
error.js:
This is the component for the error page, that is shown when an unauthorized user tries to access a page. Add the code below.
"use client" // To specify client side component
import React from 'react';
export default function ErrorPage() {
return (
<div className="flex min-h-screen items-center justify-center bg-gray-100">
<div className="w-full max-w-md p-6 bg-white rounded-md shadow-lg shadow-blue-500/50">
<div className="text-center mb-8">
<h1 className="text-4xl font-bold text-gray-800 mb-2">Oops!</h1>
<p className="text-gray-600 text-lg">Sorry, you do not have access to the requested page.</p>
</div>
<div className="flex flex-col items-center">
<img
src="https://picsum.photos/300/200"
alt="Error Illustration"
className="rounded-md w-48 h-48 mb-4"
/>
</div>
<div className="text-center">
<a href="/" className="px-6 py-2 bg-blue-500 text-white rounded-md hover:bg-blue-600 focus:outline-none focus:ring focus:ring-blue-500">
Go Back to Homepage
</a>
</div>
</div>
</div>
);
}
Building the Backend API
Create a app.js
file in the root directory i.e. my-permify-nextjs-app/app.js
and add the code below.
// Import required modules
const express = require('express');
const cors = require('cors'); // Import cors
const authorizedRoute = require('./src/api/permission'); // Import the permission middleware
const bodyParser = require("body-parser");
// Create Express app
const app = express();
// Enable CORS
app.use(cors());
app.use(bodyParser.json());
// Define routes
// Route for '/admin/:userId'
app.get('/admin/:userId', authorizedRoute('view_admin'), (req, res) => {
if (req.body && req.body.message === 'You are authorized!') {
res.json({ message: 'You have access to the dashboard!'});
} else {
res.status(403).json({ message: 'You are not authorized to access the dashboard'});
}
});
// Route for '/member/:userId'
app.get('/member/:userId', authorizedRoute('view_member'), (req, res) => {
if (req.body && req.body.message === 'You are authorized!') {
res.json({ message: 'You have access to the member page!'});
} else {
res.status(403).json({ message: 'You are not authorized to access the member page'});
}
});
// Route for '/manager/:userId'
app.get('/manager/:userId', authorizedRoute('view_manager'), (req, res) => {
if (req.body && req.body.message === 'You are authorized!') {
res.json({ message: 'You have access to the manager page!'});
} else {
res.status(403).json({ message: 'You are not authorized to access the manager page'});
}
});
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
This code sets up a web server using Express.js that handles requests for different pages (admin, member, and manager) and checks if the user has the right permission to access them. It uses a special middleware function called authorizedRoute
to check permissions.
If a user is authorized to access a page, the server sends a success message to the user; else, it sends an error message saying that the user is not authorized.
Step 2: Setting up Permify In Our Application
Permify is a powerful authorization service that helps you define and manage access control in your applications. It provides a simple way to create a central authorization service and manage user permissions.
Running Permify Service
You can run Permify Service with various options but in that tutorial we run it via docker container.
Run From Docker Container
Production usage of Permify needs some configurations such as defining running options, selecting datastore to store authorization data and more.
However, for the sake of this tutorial we'll not do any configurations and quickly start Permify on your local with running the docker command below:
docker run -p 3476:3476 -p 3478:3478 ghcr.io/permify/permify serve
This will start Permify with the default configuration options:
- Port 3476 is used to serve the REST API.
- Port 3478 is used to serve the GRPC Service.
- Authorization data stored in memory.
Test your connection
You can test your connection with creating an HTTP GET request,
localhost:3476/healthz
Defining Your Authorization Model
Permify comes with a default tenant named "t1
" that you can use directly without the need for creating a new one. This default tenant simplifies the setup process and allows you to get started quickly.
Before sending the authorization model to Permify, it's essential to understand the concepts we're modeling. In this example, we are creating a simple access control system for an organization. We'll define users and their roles within the organization, along with specific permissions for each role.
-
Entities:
user
: Represents individual users in your application.organization
: Represents the organization itself, which acts as the context for our permissions.
-
Roles: Within the organization, we define roles with different access levels:
admin
: Has the highest permissions.manager
: Has intermediate permissions.member
: Has basic permissions.
-
Permissions: Each role is assigned specific permissions that determine what actions a user can perform. In this example, we have permissions like:
view_admin
: Only admins can view content related to this permission.view_manager
: Managers and admins have access.view_member
: Members and managers have access.
Writing the Schema
Create a app-schema.js
file in the root directory i.e. my-permify-nextjs-app/app-schema.js
and add the code below.
const permify = require("@permify/permify-node");
const client = new permify.grpc.newClient({
endpoint: "localhost:3478", // Use your Permify endpoint
});
const tenantId = "t1"; // Replace with your tenant ID from Permify
async function writeSchema() {
try {
const response = await client.schema.write({
tenantId: tenantId,
schema: `
entity user {}
entity organization {
relation admin @user
relation member @user
relation manager @user
permission view_admin = admin
permission view_manager = manager or admin
permission view_member = member or manager
}
`
});
console.log('Schema written:', response);
} catch (error) {
console.error("Error writing schema:", error);
}
}
writeSchema();
Defining User-Role Relationships
To create relationships between users and their roles in Permify, you use the client.data.write()
method. This method associates a subject (a user or a role) with a specific relation within an entity.
Currently, when you try to access the admin page using an unauthorized userId named "jack", you will get an error as shown in the image below:
To fix this you will need to grant "jack
" the admin role. Then assign other roles as for follows; grant "jane
" the member role, and "john
" the manager role.
Here's how you would define these relationships:
Create a app-relationship.js
file in the root directory i.e. my-permify-nextjs-app/app-relationship.js
and add the code below.
const permify = require("@permify/permify-node");
const client = new permify.grpc.newClient({
endpoint: "localhost:3478",
})
client.data.write({
tenantId: "t1", // Replace with your tenant ID from Permify
metadata: {
schemaVersion: "cpn2bv1isfs3gfv7ke6g" // Replace with your schema version from Permify
},
"tuples": [
{
"entity": {
"type": "organization",
"id": "1"
},
"relation": "admin",
"subject": {
"type": "user",
"id": "jack",
}
},
{
"entity": {
"type": "organization",
"id": "1"
},
"relation": "member",
"subject": {
"type": "user",
"id": "jane",
}
},
{
"entity": {
"type": "organization",
"id": "1"
},
"relation": "manager",
"subject": {
"type": "user",
"id": "john",
}
}
],
"attributes": []
}).then((response) => {
// handle response
console.log(response)
})
Implementing Access Control Logic
Create a /api
folder in the /app
directory i.e. /app/api/permission.js
, then create a permission.js
file and add this code.
// Import Permify client
const permify = require('@permify/permify-node');
const client = new permify.grpc.newClient({
endpoint: "localhost:3478",
});
const authorizedUser = (permissionType) => {
return async (req, res, next) => {
try {
// Ensure req.params.userId exists
if (!req.params.userId) {
throw new Error('User ID is missing in the request parameters');
}
// Convert permissionType to string if necessary
const permTypeString = String(permissionType);
// Prepare data for Permify check request
const checkRes = await client.permission.check({
tenantId: 't1', // Replace with your tenant ID from Permify
metadata: {
schemaVersion: 'cpn2bv1isfs3gfv7ke6g', // Replace with your schema version from Permify
snapToken: '0DQC7mdS2Rc=', // Replace with your snaptoken from Permify
depth: 20,
},
entity: {
type: 'organization',
id: "1",
},
permission: permTypeString, // Use the converted permissionType
subject: {
type: 'user',
id: req.params.userId,
},
});
if (checkRes.can === 1) {
// If user is authorized
res.json({ message: 'You are authorized!' }); // Send JSON response
} else {
// If user is not authorized
res.status(401).json({ message: 'Unauthorized' }); // Send JSON response
}
} catch (err) {
console.error('Error checking permissions:', err.message);
res.status(500).json({ error: err.message }); // Send JSON error response
}
};
};
module.exports = authorizedUser;
This code defines a middleware function called authorizedUser
which checks if a user has the required permission to access a resource.
The middleware takes the permission type as an argument and uses it to check if a user, identified by their ID in the request parameters, has the required permission within the "organization
" entity with ID "1."
If the user is authorized, it sends a JSON response indicating success; else, it sends a JSON response indicating that the user is unauthorized.
Step 3: Testing your app
1. Start Permify
Ensure your Permify server is running. If you haven't setup your permify server, please visit this link to do so based on your preference.
Start Permify Server
permify serve
You should see somthing like this;
- Run your
app-tenant.js
code
node app-tenant.js
- Run your
app-schema.js
code
node app-schema.js
Copy your {schema version}
and replace it in the app-relationship.js
- Run your
app-relationship.js
code
node app-relationship.js
Copy your {schema version}
and {snaptoken}
and replace them in the permission.js
2. Start the Backend
Run your backend API
node app.js
3. Start the Frontend
Run your Next.js development server.
npm run dev
4. Log In
Go to your broswer and open localhost:3001
.
You will be shown the login page, enter the appropriate UserID based on the role and you will be redirected the role page.
Let's try to login using the "jack
" userId, that has permission to view the admin page. You will get the same result as shown in the image below;
You can play around with the different userId's to test their access to any page and see the result.
Wrap Up
You've learned how to implement access control in Next.js using permify by building a fullstack app and allowing users to login based on their roles.
Building secure web apps can be hard, but Permify makes it easier! It lets you decide who can do what in your app. It's simple to use, works great with Next.js, and is built with security in mind. This is a powerful tool for developers wanting to build secure and flexible apps.
Here's the GitHub link to gain access to the complete project.
References
To further help you understand implementation access control with Permify and Next.js, here are some valuable resources: